Creating a University Grading System Database Using SQL for College Projects
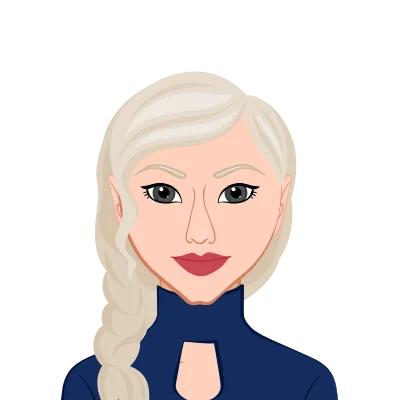
Designing and implementing a university grading system database is an essential project for computer science and IT students, offering a chance to apply theoretical concepts in a practical, real-world context. This task involves careful planning, from understanding the requirements to designing a schema, implementing it using SQL, and testing the system to ensure functionality. Whether you aim to learn database design or enhance your skills in writing complex queries, this project provides a hands-on approach to mastering SQL. One of the key aspects is understanding how to map relationships between entities like students, courses, instructors, and grades. By taking a systematic approach, you can create a robust and efficient database that reflects the intricacies of a university grading system. This guide will walk you through every step, offering insights and practical examples. If you're looking for ways to write your SQL homework effectively, this blog will be a valuable resource. It combines technical instructions with real-world scenarios to ensure a well-rounded learning experience. Dive in to explore how you can develop a database that is not only functional but also scalable and reliable, laying a strong foundation for future projects.
Understanding the Requirements of a University Grading System
To create a functional grading system, understanding its requirements is essential. This step involves identifying the key functionalities and the relationships between various entities involved, such as students, courses, instructors, and grades. A clear definition of these elements ensures a streamlined design process. If you need help with database homework tasks related to grading systems, focusing on these foundational elements will make your work much easier. This section will also explore how the database can manage data integrity and provide efficient access to stored information. By thoroughly understanding these aspects, students can build a solid foundation for their projects.
Core Functionalities
The grading system database must manage and store information related to students, courses, grades, and instructors. At its core, it should:
- Maintain student details such as names, IDs, and contact information.
- Record course offerings and associated details like course codes, names, and instructors.
- Track student enrollment in courses.
- Store and manage grades for individual homeworks, exams, and final results.
Key Relationships and Entities
To design an effective grading system, you must identify key entities and their relationships:
- Student: Includes personal and academic information.
- Course: Covers details of each course offered by the university.
- Instructor: Links instructors to their respective courses.
- Enrollment: Captures which student is enrolled in which course.
- Grade: Stores the performance metrics of students in different courses.
Understanding these entities is crucial for constructing a logical and normalized database structure.
Designing the Database Schema
Creating a database schema is a critical step in developing a grading system. This process involves identifying entities, defining their attributes, and establishing relationships. A well-structured schema lays the groundwork for a reliable and efficient database. Visual tools like ER diagrams and normalization techniques can greatly aid in this stage, ensuring the schema meets the system's requirements. This section provides a detailed walkthrough of these steps.
Entity-Relationship Diagram (ERD)
An ERD serves as a blueprint for the database, illustrating how entities interact. This diagram simplifies the design process by visually representing the structure and connections between entities. By using an ERD, students can ensure their database includes all necessary components and adheres to logical design principles.
For a grading system:
- Entities: Students, Courses, Instructors, Enrollment, Grades.
- Relationships: Students enroll in courses; instructors teach courses; grades are assigned for students in courses.
Normalization
Normalization organizes the database to minimize redundancy and maintain data integrity. By dividing data into logical tables and defining relationships, students can create a database that is easier to manage and scale. This technique also ensures that updates and deletions do not lead to data anomalies, enhancing system reliability.
For this system:
- First Normal Form (1NF): Ensure all columns contain atomic values.
- Second Normal Form (2NF): Ensure no partial dependency on a composite primary key.
- Third Normal Form (3NF): Eliminate transitive dependencies.
Implementing the Grading System Database in SQL
After designing the schema, the next step is implementing it using SQL. This involves creating tables, defining relationships, and writing SQL queries. Implementation requires attention to detail to ensure the database functions as intended. Properly defined constraints, primary keys, and foreign keys are critical for maintaining data consistency. This section demonstrates how to translate the schema into a working SQL database.
Creating the Tables
The process begins with defining tables for each entity, specifying attributes and data types. This step ensures all necessary data is captured and stored appropriately. Using meaningful names for columns and tables makes the database easier to understand and work with, especially for collaborative projects. Below is a step-by-step guide:
1. Student Table
CREATE TABLE Student (
StudentID INT PRIMARY KEY,
FirstName VARCHAR(50),
LastName VARCHAR(50),
Email VARCHAR(100),
PhoneNumber VARCHAR(15)
);
This table stores the details of each student, ensuring each has a unique StudentID.
2. Course Table
CREATE TABLE Course (
CourseID INT PRIMARY KEY,
CourseName VARCHAR(100),
Credits INT,
InstructorID INT
);
The Course table includes course-specific information, linking to the instructor responsible for the course.
Establishing Relationships
Defining relationships between tables using foreign keys ensures data integrity. These relationships allow for meaningful connections between data, enabling complex queries and accurate data retrieval. This section shows how to implement these relationships with SQL commands, providing a complete picture of the database structure.
3. Instructor Table
CREATE TABLE Instructor (
InstructorID INT PRIMARY KEY,
FirstName VARCHAR(50),
LastName VARCHAR(50),
Email VARCHAR(100)
);
This table manages instructor details. InstructorID acts as the foreign key in the Course table.
4. Enrollment Table
CREATE TABLE Enrollment (
EnrollmentID INT PRIMARY KEY,
StudentID INT,
CourseID INT,
Semester VARCHAR(10),
Year INT,
FOREIGN KEY (StudentID) REFERENCES Student(StudentID),
FOREIGN KEY (CourseID) REFERENCES Course(CourseID)
);
The Enrollment table establishes the many-to-many relationship between students and courses, using foreign keys.
5. Grade Table
CREATE TABLE Grade (
GradeID INT PRIMARY KEY,
EnrollmentID INT,
HomeworkScore DECIMAL(5,2),
ExamScore DECIMAL(5,2),
FinalGrade DECIMAL(5,2),
FOREIGN KEY (EnrollmentID) REFERENCES Enrollment(EnrollmentID)
);
The Grade table links scores to specific students enrolled in courses, using the EnrollmentID foreign key.
Populating the Database with Sample Data
Populating the database with sample data is essential for testing and demonstrating its functionality. This step allows students to verify their implementation and debug any issues. By inserting realistic data, they can simulate real-world scenarios and assess the database's performance under various conditions. This section includes examples of how to populate the database and write SQL queries to retrieve meaningful insights.
Inserting Data into Tables
Adding data to the tables involves using SQL INSERT statements. Careful consideration of data integrity and adherence to constraints is crucial during this step. This section provides examples of inserting data into each table, ensuring the database is ready for testing.
Student Table
INSERT INTO Student (StudentID, FirstName, LastName, Email, PhoneNumber)
VALUES (1, 'John', 'Doe', 'john.doe@example.com', '1234567890');
Course Table
INSERT INTO Course (CourseID, CourseName, Credits, InstructorID)
VALUES (101, 'Database Systems', 3, 1);
Using Joins to Retrieve Data
Joins are powerful SQL features that combine data from multiple tables. They allow for complex queries, such as retrieving students' grades for specific courses. This section demonstrates how to write effective join queries, enabling students to unlock the full potential of their database. For instance, to get students’ grades for a course:
SELECT S.FirstName, S.LastName, C.CourseName, G.FinalGrade
FROM Student S
JOIN Enrollment E ON S.StudentID = E.StudentID
JOIN Grade G ON E.EnrollmentID = G.EnrollmentID
JOIN Course C ON E.CourseID = C.CourseID
WHERE C.CourseName = 'Database Systems';
This query links Student, Enrollment, Grade, and Course tables to retrieve the desired information.
Advanced Features and Optimization
Advanced features and optimization techniques enhance the database's functionality and performance. Automating repetitive tasks and improving query efficiency ensures the system runs smoothly. This section explores features like triggers and indexing, which add value to the database and make it more robust. These techniques are especially useful for large datasets and high-traffic environments.
Automating Grade Calculation
Automation reduces manual effort and improves accuracy. Triggers can be used to calculate final grades automatically based on predefined formulas. This section provides an example of creating a trigger to compute grades, demonstrating how automation can simplify processes.
To automate final grade calculations, use a trigger:
CREATE TRIGGER CalculateFinalGrade
BEFORE INSERT ON Grade
FOR EACH ROW
BEGIN
SET NEW.FinalGrade = (NEW.HomeworkScore * 0.4) + (NEW.ExamScore * 0.6);
END;
This trigger ensures FinalGrade is computed automatically based on predefined weightage.
Indexing for Performance
Indexing speeds up query execution by reducing the time required to locate data. This is particularly beneficial for frequently searched columns. This section explains how to create indexes and measure their impact on query performance, helping students optimize their database effectively. To speed up queries, create indexes on frequently searched columns:
CREATE INDEX idx_student_name ON Student (FirstName, LastName);
CREATE INDEX idx_course_name ON Course (CourseName);
Testing and Debugging the System
Testing and debugging are crucial for ensuring the database operates as expected. By simulating various scenarios, students can identify and resolve issues, enhancing the system's reliability. This section covers common test cases and debugging tips to help students refine their database and achieve optimal performance.
Common Test Cases
Testing involves verifying that the database handles all required operations correctly. This includes enrolling students, assigning grades, and retrieving reports. Identifying edge cases and validating foreign key constraints ensures the database remains consistent and error-free.
Debugging Tips
Debugging involves analyzing errors and improving the database's design or queries. Tools like EXPLAIN can help identify inefficiencies in query execution. This section provides practical tips for debugging, enabling students to address issues effectively and enhance their database's performance.
Conclusion
Creating a university grading system database involves a blend of thoughtful design and technical SQL implementation. By following the steps outlined in this guide, you can build a robust and efficient database system suitable for college projects. This hands-on approach not only helps in solving homeworks but also equips you with valuable skills for real-world database design.